前回
前回のブログでは、TensorFlow Lite for microのサンプル(Hello world)をM5StickV向けにビルド、動作させるところまでを書いた。出力はコンソール出力だけで、M5StickVのLCDには何も表示しない。
目的
今回は、M5StickVのLCDに表示を行うところをメインにする。
こんな感じである。
参考
LCDに表示するまでの道のり
LCDに表示するにはHWの制御がかなり必要である。自前でやるのもよいが、今回はsipeedが提供するMaixPyに含まれるライブラリを使用することにする。
前回のプロジェクトに追加・変更する方針とする。
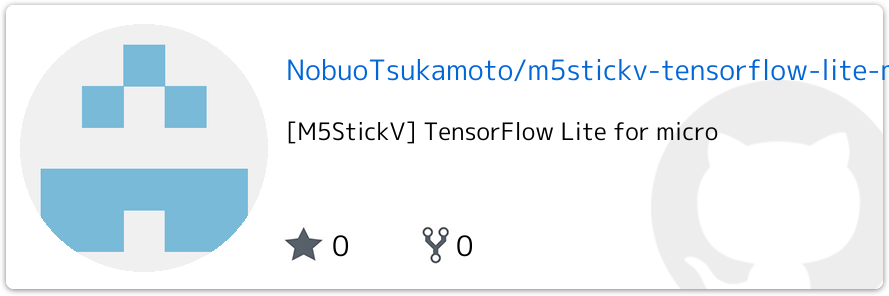
sipeed / MaixPyのMaixPyのSDK
もともと、Micropython 用の環境であるが、SDKと利用して独自のcプロジェクトをビルドすることができる。
ただ、C++はビルドできないため、SDKのみを利用することにした。
components配下のSDKの部分を利用する。以下のフォルダを利用した。
- boards
- drivers
- kendryte_sdk
- utils
sipeed/MaixPyのSDKをプロジェクトに追加
プロジェクトに追加する。
- sipeed/MaixPyのSDK(compoents配下)をlib(kendryte K210のStandard SDK)配下に配置。
- CMakeLists.txtにcompoentsを加えるように変更。
(Githubの差分はこちら)
sipeed/MaixPyのSDKをC++から呼び出せるように変更
sipeed/MaixPyのSDKはC++から呼び出すことを考慮していない箇所が多数ある。このため、使用する部分のヘッダを変更する。
extern "C" の追加し、呼び出し可能とする。
今回は以下のヘッダを修正。
TensorFlow lite for microのソース変更
以下の処理を追加する。
- main.ccでLCDを含むHWの初期化
- output_handler.ccで描画の更新
HWの初期化
src/micro/tensorflow/tensorflow/lite/micro/examples/hello_world/main.ccで行う。
- HWの初期化を行う。
m5stick_init()
(BUTTON B長押しの電源OFFもできるようになったりする) - setup_lcd()でLCD初期化を行う。
man.ccはこのような感じになる。
出力値に応じた点の描画
STM32F746の実装を参考。LCD出力用の関数をsipeed/MaixPyのSDKを使用する。ただ、元の実装では円を描画しているが、sipeed/MaixPyのSDKには用意されていない。今回は矩形描画で代替えした。
使用した関数は以下。
- lcd_get_width ... LCDの幅を取得
- lcd_get_height ... LCDの高さを取得
- lcd_clear ... LCDのクリア
- lcd_fill_rectangle ... 矩形を描画
output_handler.ccはこのような感じになる。
最後に
前回、今回とM5StickVでTensorFlow Lite for microのサンプルをビルドするやり方を記載した。M5StickVには6軸センサやカメラがあるので、他のサンプルも行けると思うのでチャレンジしてみたい。
0 件のコメント:
コメントを投稿